Blending The Alpha!
Well, I had this issue and it annoyed me a lot.
The Issue was very easy to comprehend. There is something wrong with the Alpha!
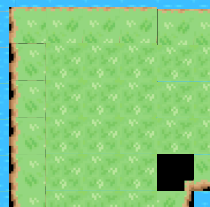
But the Solution? For a newbie I thought the issue was in the DDS Texture. The texture raised suspicion because I had just blindly selected the ARGB 1 bit alpha.
So the doubt was:
1) Is the texture flawed?
2) Is there something missing in the Code?
And yeah, There was something missing in the code indeed. The texture generated from NVIDIA Texture tools were correct.
Here is the Final Output:
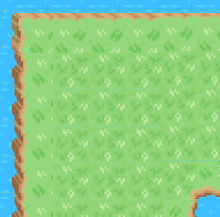
So what did the Magic? It was: BlendingState (ID3D11BlendState) which needed to be set on how Layers of the TileObject are Blending with the each other.
This Gist explains the 3 files that I changed to add Blending for Alpha.
World of Floating Point
So I hooked up a small setup of Map Generation in Azura and got the following render.
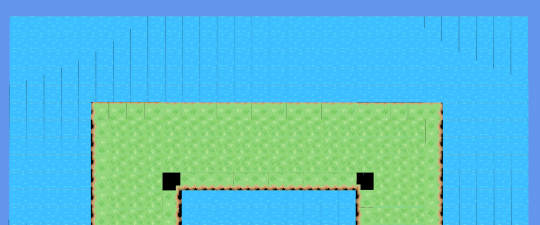
Notice the black lines? I think these black lines are due to the floating point values assigned as the texture coordinates. As only the texture map has black backgrounds.
If I move the map somehow the lines change. This may be because the camera has a tilted view towards some of the tiles causing them to be rendered this way? Here is another screenshot of the camera moved a bit to the left:
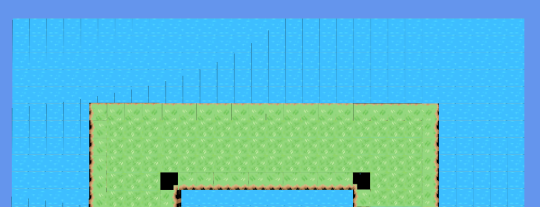
Notice how the black lines are now at the center? .. Weird … Investigation going on!
Resource Management
Intitally all the code was in the Main.cpp for my Game for loading resources. This had to be moved and there was a need to make a ResourceManager that would be capable of using the existing Loader class to load textures, files etc. all async.
Taking Azura UI to the next level
While implementing the UI functionality of Azura, I faced a little problem in segregating code for Different HUDs like Settings related UI Elements should be in a Settings related class and same goes for Main Menu Elements, Levels UI Elements etc.
But it was not possible with the Current strucutre. So I moved things around.
I coded an abstract class called Scene.
ref class Scene abstract { internal: Scene( ID2D1Factory3* d2dFactory, IDWriteFactory3* dwriteFactory, ID2D1DeviceContext2* d2dContext, CoreWindow^ window ); // Override Functions (Hooks) virtual void Init() = 0; virtual void Update() = 0; virtual void OnSceneEnter() = 0; virtual void OnSceneExit() = 0; // Internal Common Functions UIHUD^ CreateHUD(std::wstring id); // Direct 3D Render and Update Phase void UpdatePhase(); void RenderPhase(D2D1::Matrix3x2F orientationMatrix); std::map<std::wstring, UIHUD^> GetHUDMap() { return m_hudMap; } // Other Utilities CoreWindow^ GetWindow() { return m_window; } UIHUD^ GetHUD(std::wstring id); private: CoreWindow^ m_window; std::map<std::wstring, UIHUD^> m_hudMap; Microsoft::WRL::ComPtr<ID2D1Factory3> m_d2dFactory; Microsoft::WRL::ComPtr<IDWriteFactory3> m_dwriteFactory; Microsoft::WRL::ComPtr<ID2D1DeviceContext2> m_d2dContext; };
What’s so awesome is that I am now able to create Scenes seamlessly. Also, Each scene has now a collection of HUDs (earlier there was a UIManager, which has now been deprecated).
So who does the gluing of all the Scenes ? Answer: The SceneDirector.
ref class SceneDirector sealed { internal: SceneDirector(); void AddScene(std::wstring id, Scene^ scene); void ChangeScene(std::wstring id); Scene^ GetScene(std::wstring id); void DeleteScene(std::wstring id); void Update(); void Render(D2D1::Matrix3x2F oritentationMatrix); void Clear(); private: Scene^ m_currentScene; std::map<std::wstring, Scene^> m_sceneMap; };
The director stores the Scenes beautifully in std::map<std::wstring, Scene^> m_sceneMap. This enables me to define Scenes as:
ref class MainMenuScene sealed : public Scene { internal: MainMenuScene( ID2D1Factory3* d2dFactory, IDWriteFactory3* dwriteFactory, ID2D1DeviceContext2* d2dContext, CoreWindow^ window ); void Init() override; void Update() override; void OnSceneEnter() override; void OnSceneExit() override; };
Here I created a custom MainMenuScene which stores all the UI Elements only specific to the Main Menu. This way I can create multiple Scenes and store them in individual files and reusing a lot of code. Also I didn’t use the interface attribute for the Scene class because I wanted to create some common functions like createHUD() which is not possible when making an Interface like IScene as all the methods have to be pure virutal there.
Saturday Hack Day - Azura
Been working on my Game. I finally got about 2-3 hours free to quickly hack a GUI Manager for the game. Oh boy, It works amazing as hell.
I initialize it as:

So I can now create HUDs which in-turn consists of Buttons and Basic UI Blocks. I have also added a CanRender() and CanUpdate() to each of them so It is easy to control what elements are to be visible in the HUD at a given time. Also the UIManager controls all the HUDs and plays a key role in rendering only those HUDs which are render-able (HUDs also have their own CanRender() :) ), This way I can have Multiple HUDs faster and cleaner.
This is how the Inheritance is flowing:
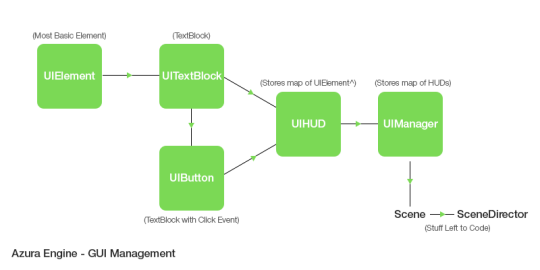
Wait there is still stuff left!
I have yet to code the Scene and SceneDirector, So for eg:
Settings Scene
- will have 1 UIManager
- will have multiple HUDs
- each HUD will correspond to a Particular Setting say Display, Sound etc.
The SceneDirector will be responsible for loading the Scene “Settings,” this scene will probably inherit from an Interface (IScene) and SettingsScene will have the Click Events and other Related variables (like strings etc) for that Scene only.
Sounds a bit complex but easy to Implement if given a thought.
Been on a long code sprint. Hoping to get some free time for my Game soon.
Been working on RequireJS and loading apps dynamically through PouchDB and Electron. It’s amazing as hell ! :)
Game Plan
Planning is important before I jump into coding my Game. Here is a list of stuff that I have prepared to make my game and center it around a theme/seed.
Seed for my Game: Stone
I watched in a recent GDC Talk that it is important to center your game around a theme. Also being my first game it also has to have a very limited scope. Something which is small and fun.
Yes Stone. The entire game will be pretty basic. About a 3 unit turn based strategy game. Simple and easy to use. I will center the game on random terrain generation (ScaledJS will be converted to ScaledC :) ) and provide same screen multiplayer for Couch Co-op gaming something similar to worms.
Think of it as: Small Scale Civilization V + Worms
Will need to make it gamepad compliant since it will be a couch multiplayer game.
Well now that I have my theme and idea. I need a set of tasks and a set of deadlines. Given that I am working at my company (StoreDock) and I am also involved in many projects other than this, I need a good schedule that can fit my busy workload.
Basic Initial Tasks
- Game Assets - Buttons and basic UI
- Stone like Buttons for Start Menu, Game UI and Pop Ups
- Game Textures - Tiles & basic Unit Textures
- Tiles: Grass, Water, Forest
- Units: Swordsman, Archer, Catapault, Horse
- Buildings: Castles (Occupied and Unoccupied)
- ScaledJS port to ScaledC
- Port all needed APIs to C
- or Find out a way to called ScaledJS from C++/CX
- Upgrading Azura - State and State Manager
- Using Azura’s State Manager (Made one recently need to test)
- Upgrading Azura - Units, Melee Units, Ranged Units
- Ability to Define Units just like Tiles
- Upgrading Azura - Movement Control
- Support for Keyboard
- Support for Mouse
- Support for X360 Controller
- Game Logic - Combat System
- Decide the Combat Bonuses based on Terrain
- Game Logic - Health System
- Regeneration
- Poison / Decay HP (Add Support not needed in Game)
- Game Logic - Unit Movement System
- Ability to Decide Movements using A* Algorithm
- Game Logic - Basic Utils like Game Start, End etc.
Introducing Azura
Yes. My engine’s name is based of one of Skyrim’s Daedric Princes, Azura. Now that’s settled, I have been working on a Game / Game Engine on DirectX. This is probably my first attempt towards at creating a game.
Why Azura? Well … so I will just throw in a picture of Azura.
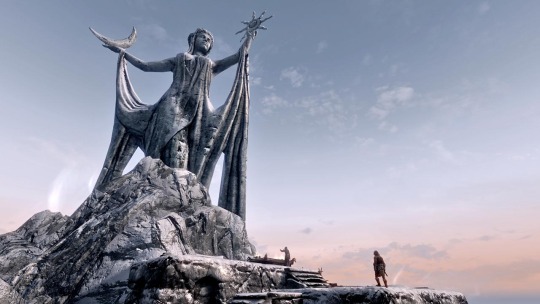
Why not use Unity / Cocos ?
Well I am very proficient in JavaScript and Cocos2D JS + ScaledJS seems to be a very comfortable side. But the problem comes when you use Cocos 2DJS you are abstracted on a whole new level. Cocos 2DJS, even 2DX have made proper abstractions over the OpenGL environment.
Which is good for:
- Rapidly Making Games
- Not worrying about what is going on underneath?
Which is not good for:
- When you want to make your own engine
- When you want a deeper integration with device level APIs
Sure making games is awesome and fast on Cocos but I really want to learn the way actual desktop games are made which involves using Direct3D or OpenGL Graphics Library directly.
Sure the path is hard. But it is just a start that I wanted.
I will update my progress on how the Game goes :)